An Introduction to Gradient Descent
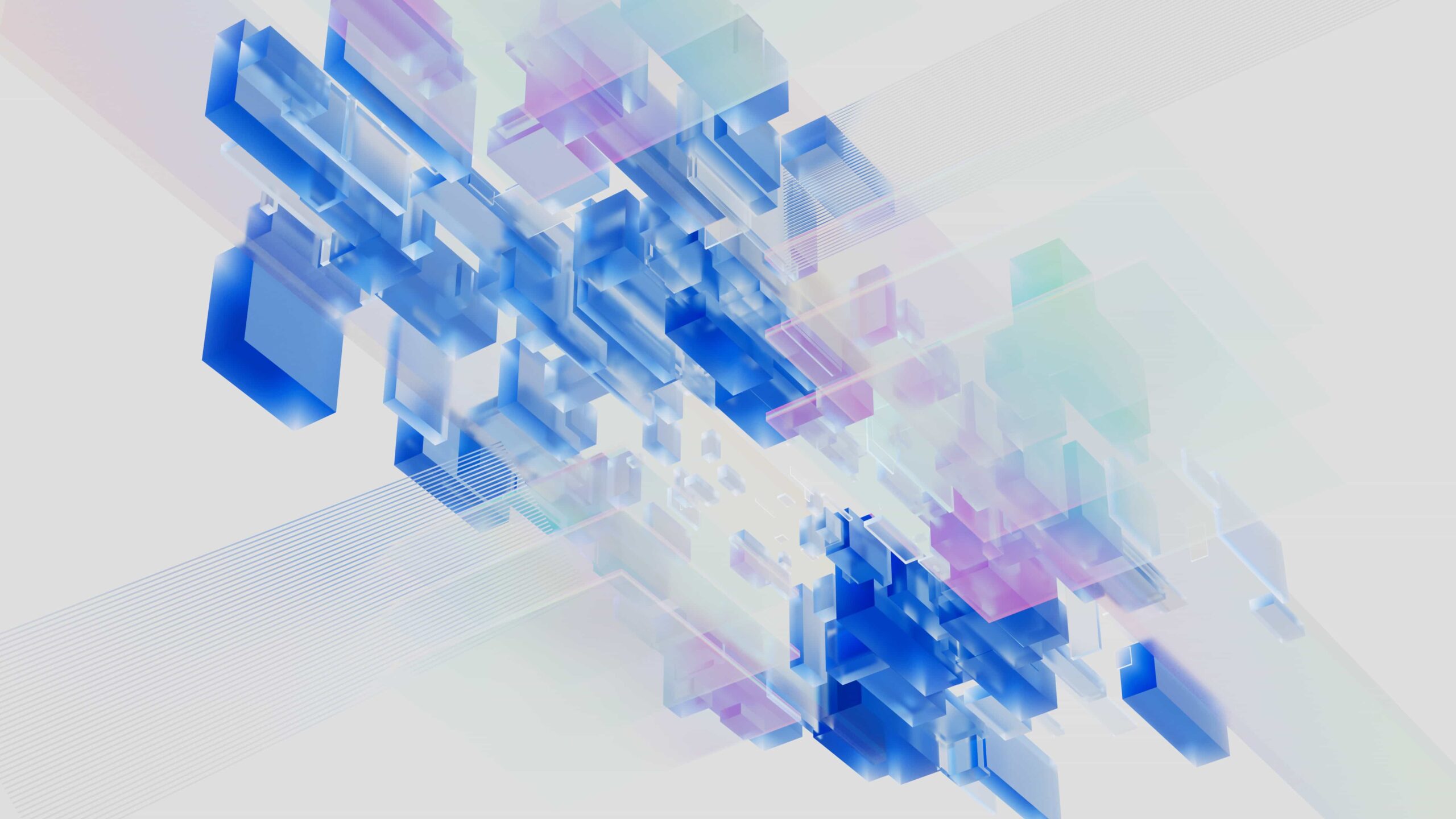
What is Gradient Descent?
Gradient descent is an optimization algorithm that is commonly used to minimize cost functions and train machine learning models. It works by taking steps in the direction of steepest descent, which means moving towards lower values of the cost function. The basic idea is that we start with some initial parameter values, compute the gradient of the cost function with respect to the parameters, and move in the opposite direction of the gradient since we want to minimize the cost. This process is repeated iteratively until the algorithm converges to a minimum.
Gradient descent is used extensively in deep learning and neural networks to optimize the weights and biases of the network by minimizing a loss function. It is also used in many other machine learning algorithms like linear and logistic regression.
Some key properties of gradient descent:
- Iterative algorithm – takes multiple steps to reach optimum
- Guaranteed to converge to local minimum for convex problems
- Efficient for high-dimensional parameter spaces
- Simple to understand and implement
So in summary, gradient descent starts with a random set of parameter values, calculates the gradient of the cost function, and updates the parameters in the negative gradient direction to minimize the cost function iteratively. It is one of the most popular optimization algorithms used in machine learning.
How Does Gradient Descent Work?
The working of gradient descent is fairly straightforward but involves some intricate mathematics. Let’s break it down step-by-step:
- Initialize parameters (theta): Choose random starting values for the parameters. eg. weights and biases in a neural network.
- Calculate gradient: Compute the gradient of the cost function with respect to all parameters. The gradient indicates the direction of steepest ascent.
- Update parameters: Update each parameter by moving in the opposite direction of the gradient by taking a small step size (learning rate). theta = theta – learning_rate * gradient
- Repeat steps 2 and 3: Calculate gradients and update parameters iteratively until convergence when gradient is very small.
The learning rate is a hyperparameter that controls how big of a step we take in the negative gradient direction. A larger learning rate leads to faster convergence but can overshoot the minimum. A smaller learning rate is more precise but takes longer to converge.

Math Behind Gradient Descent
The math behind gradient descent utilizes calculus and derivatives to determine the gradients. Let’s break it down:
- We define a cost function J(theta) that we want to minimize.
- To minimize it, we calculate the partial derivatives with respect to each parameter theta_i: dJ/d(theta_i)
- The gradient is the vector of all the partial derivatives. It points in the direction of steepest ascent.
- To minimize J, we update each theta_i by moving in the opposite direction of the gradient: theta_i = theta_i – learning_rate * dJ/d(theta_i)
This mathematical basis of calculating gradients and updating parameters enables gradient descent to minimize the cost function.
Types of Gradient Descent
There are three variants of gradient descent that are commonly used:
1. Batch Gradient Descent
In batch gradient descent, the gradient is computed over the entire training dataset before the parameters are updated. This means we have to do a forward and backward pass over ALL the training examples before updating the parameters once. Batch gradient descent is very slow and infeasible for large datasets. But it guarantees convergence to the global minimum for convex error surfaces and is more stable.
2. Stochastic Gradient Descent
Stochastic gradient descent (SGD) updates the parameters for each training example. This means the parameters are updated after computing the gradient for just ONE training example. SGD is much faster and convenient for large datasets. However, it introduces noise in the gradient and may never converge to the minimum due to fluctuations.
3. Mini-Batch Gradient Descent
Mini-batch gradient descent takes the best of both worlds – it performs an update for every small batch of n training examples. Typical batch sizes range from 2 to 100s. This balances computation speed and convergence stability. In practice, mini-batch SGD is most commonly used in deep learning.
The batch size is a key hyperparameter than needs to be tuned properly for good performance. Smaller batch size has more noise in gradient but can find minimum quicker. Larger batch size has low noise but converges slowly.
Challenges with Gradient Descent
Despite its simplicity and popularity, gradient descent has some challenges:
- Choosing learning rate can be difficult. A suboptimal learning rate could lead to slow convergence or no convergence.
- It can get stuck in local minimas when the error surface is non-convex.
- Flat regions and plateaus can cause very small gradients, making progress very slow.
- Sensitive to feature scaling. Features on different scales cause convergence issues.
- Finding global minimum is not guaranteed for non-convex problems.
Many techniques have been developed to address these challenges like momentum, adaptive learning rate, mini-batch SGD, etc. Careful tuning of hyperparameters is crucial for good performance.
Applications of Gradient Descent
Some important applications where gradient descent shines are:
- Training deep neural networks for computer vision, NLP and more. It is the workhorse for optimizing network weights.
- Training linear and logistic regression models for prediction tasks. It minimizes the loss function.
- Training support vector machines where it maximizes the margin between classes.
- used in recommender systems to minimize prediction error.
- Finding optimal parameters in regularization techniques like LASSO and Ridge regression.
So gradient descent is applicable to a vast range of machine learning algorithms and models like regressors, classifiers and neural networks. It is the backbone of modern deep learning.
Gradient Descent Implementation in Python
Let’s see a simple gradient descent implementation for linear regression in Python:
import numpy as np
# training data
X = np.array([[1,1], [2,2], [3,3]])
y = np.array([1, 2, 3])
# initialize parameters
theta = np.array([0, 0])
# gradient descent settings
iterations = 1000
alpha = 0.01
m = len(y)
for i in range(iterations):
# compute gradient
gradient = (2/m) * np.dot(X.T, (np.dot(X, theta) - y))
# update parameters
theta = theta - (alpha * gradient)
print(theta)
# sample output
# [0.99935587 0.99933838]
Here we implemented a simple linear regression model with gradient descent optimization and decent parameter convergence. This can be extended to other algorithms as well.
Proper data preprocessing, feature engineering and hyperparameter tuning is crucial to ensure gradient descent works well. Libraries like TensorFlow and PyTorch provide auto differentiation capabilities so you don’t have to write the gradient calculations manually.
Summary
In summary, gradient descent is a simple yet powerful optimization algorithm that is driving machine learning today. Key takeaways:
- Used to minimize cost functions and train models by iteratively moving in the direction of steepest descent
- Computes gradient of cost function and updates parameters in the negative gradient direction
- Comes in three flavors – batch, stochastic and mini-batch gradient descent
- Has some challenges like local optima, learning rate selection etc.
- Crucial role in training neural networks and many other ML algorithms
- Fairly straightforward to implement in Python and other languages