Demystifying Hidden Markov Models: A Beginner’s Guide
Unlock the power of Hidden Markov Models (HMMs) with this comprehensive beginner's guide. Learn what HMMs are, how they work, and their applications.
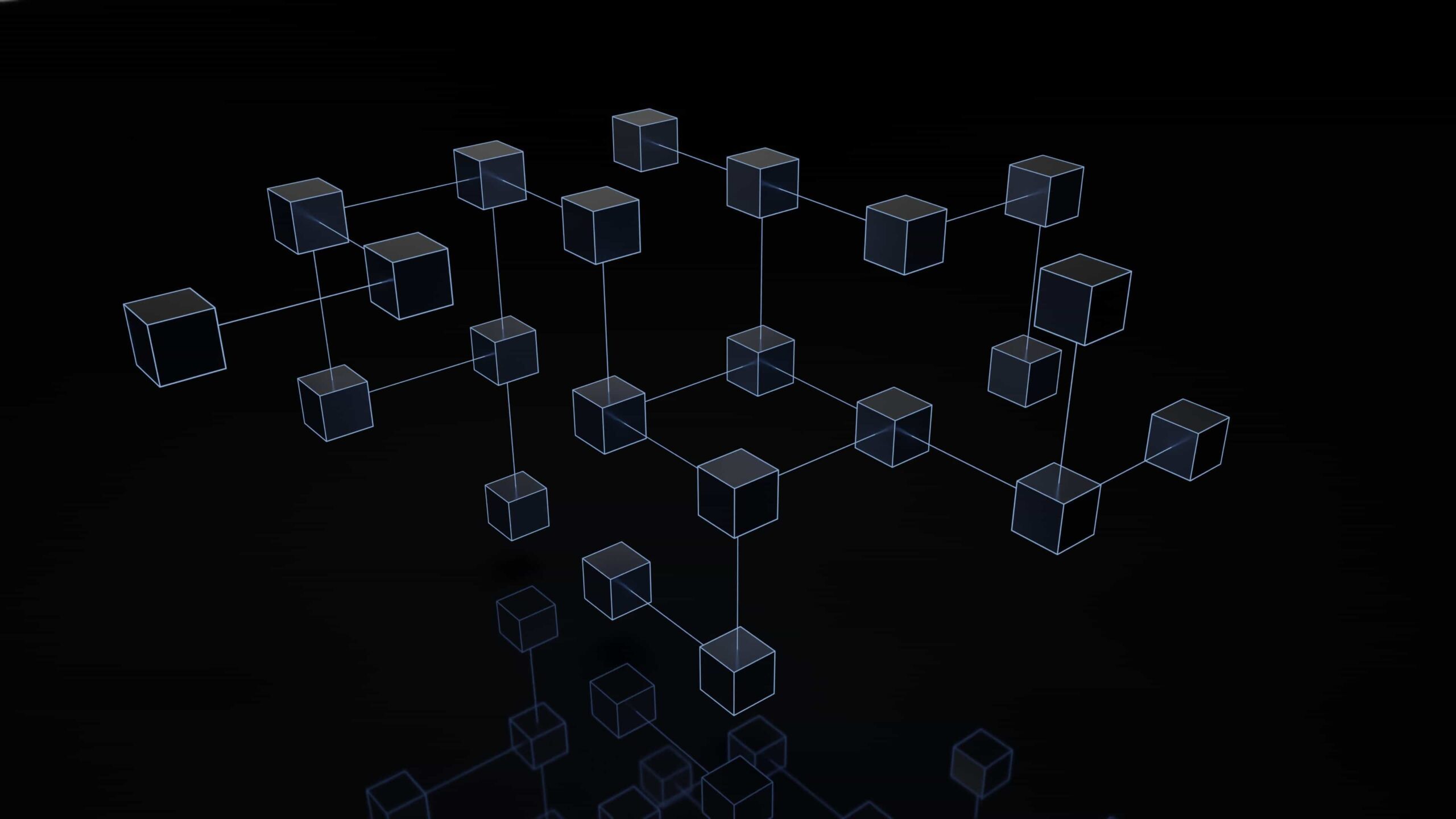
Introduction to Hidden Markov Models
Hidden Markov models (HMMs) are a powerful statistical tool for modeling sequential and time series data. Though complex in theory, HMMs have widespread applications in fields like speech recognition, natural language processing, and bioinformatics. In this comprehensive beginner’s guide, we will demystify HMMs by explaining what they are, how they work, their applications, and how to implement them in Python.
What is a Hidden Markov Model?
A hidden Markov model is a statistical Markov model where the system being modeled is assumed to be a Markov process with unknown parameters. The challenge is to determine the hidden parameters from the observable parameters. HMMs have two components – an underlying, unobservable Markov chain with hidden states, and a set of observable output dependent on those states.
In simpler terms, a HMM models a system where the state is hidden, but the output is visible. The model determines the hidden state sequence that best explains the observed output sequence. The ‘hidden’ in HMM refers to the state sequence and not the output. HMMs assume the current state only depends on the previous state, satisfying the Markov property.
Why are they called ‘Hidden’?
HMMs are called hidden because the internal state sequence generating the outputs is not known or ‘hidden’. Only the outputs corresponding to those hidden states are visible. The task is to estimate the most likely state sequence given the output sequence. The ‘hidden’ states are the inner workings of the model that generate the visible outputs.
Key Components of a HMM
A HMM is characterized by three key components:
- Hidden states – The internal/hidden states (S) that generate the outputs.
- Output probabilities – Probability of observable outputs (O) given hidden states.
- Transition probabilities – Probability of transitioning from one hidden state to another.
These components allow HMMs to model complex real-world sequences and systems. By modeling state transitions and output probabilities, HMMs can uncover hidden patterns.
HMM Representation
The components of a HMM can be formally defined as:
- S = {S1, S2…SN} – The set of N hidden states
- O = {O1, O2…OM} – The set of M observable output symbols
- A = State transition probabilities
- Ai,j = P(Sj at t+1 | Si at t)
- B = Output probabilities
- Bj(k) = P(Ok | Sj)
- π = Initial state probabilities
- πi = P(Si at t=1)
This compact representation captures the complete specification of a HMM. The matrices A, B, π fully define the model.
How Do Hidden Markov Models Work?
Now that we have defined a HMM, let’s understand how they work in action. The key steps in HMMs are:
1. Model Initialization
The model parameters A, B, π are initialized based on domain knowledge or randomly. This gives starting estimates for the hidden states and their output/transition probabilities.
2. Forward-Backward Algorithm
This key step efficiently computes the posterior probabilities of state sequences given observed outputs via dynamic programming. It re-estimates the model parameters to best fit the observed data.
3. Decoding
The Viterbi algorithm finds the single best state sequence by maximizing the joint probability of the sequence and observed outputs. This gives the most likely explanation of the hidden states.
4. Evaluation
The forward-backward probabilities are used to evaluate model accuracy and select the optimal HMM architecture. Thresholds determine when re-estimation should stop.
By repeating steps 2-4, HMM parameters are iteratively refined to uncover the hidden structure in the data. These steps enable HMMs to decode complex sequential phenomena.
Key Applications of Hidden Markov Models
Due to their sequence modeling capabilities, HMMs have become ubiquitous across domains, with some major applications being:
Speech Recognition
HMMs are extensively used in speech processing to model spoken words and languages. The hidden states represent sub-phonetic units while the outputs are spectral audio features. HMMs can recognize speech despite variability across speakers.
Gesture Recognition
Computer vision systems use HMMs to model human gestures in video sequences. The hidden states correspond to gesture primitives while the observable outputs are hand movements and poses. HMMs can robustly recognize gestures.
Gene Prediction
Bioinformatics applications employ HMMs for modeling DNA and protein sequences to identify genes. The hidden states represent exons, introns, intergenic regions while outputs are sequence motifs like start/stop codons.
Part-of-Speech Tagging
HMMs tag words in sentences with their correct part-of-speech based on lexical context and grammar. The hidden states are the POS tags while the outputs are the observed words. HMMs outperform rule-based POS taggers.
Activity Recognition
Wearable sensors use HMMs to identify human activities like walking, running, sitting etc. The hidden states represent activities while outputs are sensor readings. HMMs can detect activities from sensor data.
These demonstrate the diversity of systems that HMMs can model. Their ability to capture temporal context and sequence phenomena has led to widespread adoption.
HMM Algorithms Explained
Now let’s delve into the key algorithms that empower HMMs:
1. Forward Algorithm
The forward algorithm efficiently computes the forward probability α(i,t) – the probability of observing the first t outputs and being in state Si at time t, given the model parameters.
It uses dynamic programming to combine probabilities of previous states, avoiding exponential computations. The forward probabilities can be used to determine the likelihood of an observed output sequence.
2. Backward Algorithm
Similarly, the backward algorithm computes the backward probability β(i,t) – the probability of observing outputs from t+1 to T given state Si at time t.
It recursively computes β in a backward manner starting from the end of the sequence. The backward probabilities are used alongside the forward variables to re-estimate model parameters.
3. Baum-Welch Algorithm
The Baum-Welch algorithm uses the forward and backward probabilities to re-estimate the model parameters A, B, π. It iteratively maximizes the probability of observed sequences to determine the HMM parameters that best fit the data.
4. Viterbi Algorithm
The Viterbi algorithm computes the most likely state sequence by maximizing the joint probability of hidden states and observed outputs. This decoding gives the optimal explanation of the hidden states.
These interlinked algorithms enable HMMs to model complex real-world sequences and uncover the hidden structures within them.
Implementing HMMs in Python
HMMs can be implemented in Python using libraries like hmmlearn, pomegranate, and PyMC. Here is a quick example:
python
# Import and initialize HMM
import hmmlearn.hmm as hmm
model = hmm.GaussianHMM(n_components=3)
# Fit on training data
model.fit(X_train)
# Get most likely state sequence for observations
state_sequence = model.predict(X_test)
# Evaluate model performance
model.score(X_test)
The hmmlearn package provides an easy API for training, predicting, and evaluating HMMs in Python. With just a few lines of code, you can start uncovering hidden states from observable data using HMMs.
Conclusion
This brings us to the end of this comprehensive guide to Hidden Markov Models. We covered the intuition, mathematics, algorithms, applications and implementation of HMMs. The key takeaways are:
- HMMs model hidden states and observable outputs via transition and emission probabilities.
- Forward, backward, Baum-Welch, and Viterbi algorithms drive learning and inference in HMMs.
- HMMs are applied extensively in speech, vision, NLP, and bioinformatics problems.
- Python libraries like hmmlearn make it easy to use HMMs.
HMMs are immensely useful for sequential data modeling. I hope this tutorial helped demystify them for you. Happy learning!