Implementing K-Means Clustering: A Beginner’s Guide to Unsupervised Learning
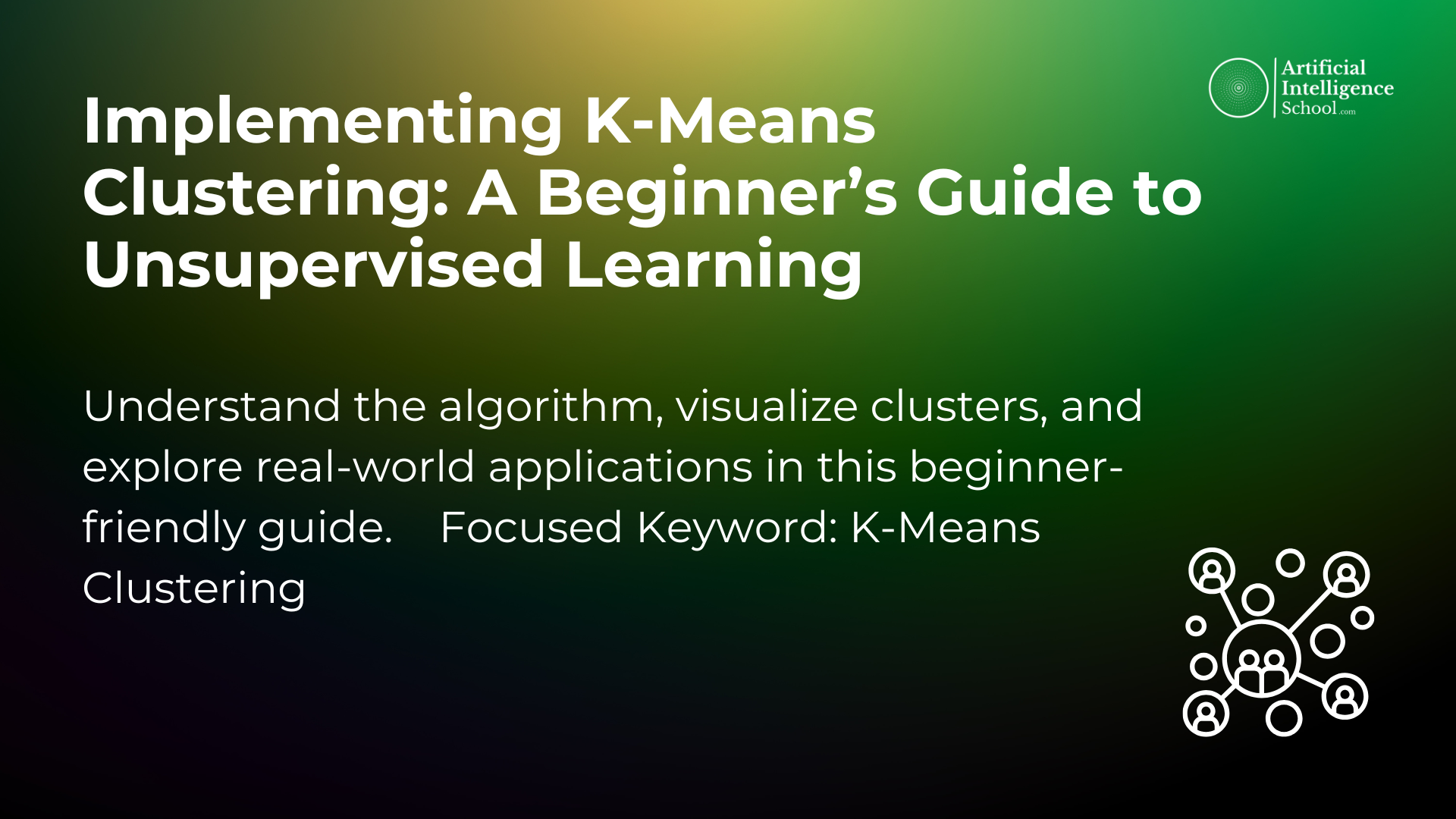
Introduction to Unsupervised Learning
Unsupervised learning is a category of machine learning where algorithms learn patterns from data without any labeled outcomes or explicit instructions on what to predict (Supervised vs. Unsupervised Learning: What’s the Difference? | IBM). In other words, the model isn’t given “right answers” during training – it must find structure in the data on its own. This is important because in many real-world scenarios, labeled data is scarce or expensive to obtain, and we still want to discover meaningful insights from raw data. By uncovering hidden patterns or groupings, unsupervised learning can reveal insights that might not be immediately obvious, helping analysts explore data and make informed decisions.
Types of Unsupervised Learning: Common unsupervised learning tasks include:
- Clustering: Grouping similar data points into clusters based on their features (e.g. K-Means, hierarchical clustering). This is useful for discovering categories or segments in data (Supervised vs. Unsupervised Learning: What’s the Difference? | IBM).
- Dimensionality Reduction: Simplifying data by reducing the number of features while preserving important structure (e.g. PCA, t-SNE). This helps visualize data or speed up algorithms by removing noise and redundancy (Supervised vs. Unsupervised Learning: What’s the Difference? | IBM).
- Association Rules: Finding relationships in data (e.g. market basket analysis where you discover that customers who buy item A often also buy item B) (Supervised vs. Unsupervised Learning: What’s the Difference? | IBM).
- Anomaly Detection: Identifying outliers or unusual data points that don’t fit established patterns (useful for fraud detection, fault detection, etc.) (Supervised vs. Unsupervised Learning: What’s the Difference? | IBM).
Each of these methods serves a different purpose, but they all share the characteristic of learning from unlabeled data. In this tutorial, we will focus on clustering, and in particular, the popular K-Means clustering algorithm.
Overview of K-Means Clustering
What is K-Means? K-Means is a widely-used centroid-based clustering algorithm that partitions data into K distinct clusters (What is k-means clustering? | IBM). The “K” in K-Means is the number of clusters you want the algorithm to find. It’s an unsupervised method, meaning it figures out how to group the data without any predefined labels. K-Means is popular for its simplicity and efficiency in discovering group structure in data. It’s used in many real-world applications such as customer segmentation, image compression, and document clustering (What is k-means clustering? | IBM).
How K-Means works: At its core, K-Means tries to place cluster centers (called centroids) such that data points assigned to each centroid are as close as possible to it. The algorithm works iteratively in the following steps (Stop Using Elbow Method in K-means Clustering | Built In):
- Choose K: Decide on the number of clusters K for your data. (This is a crucial parameter that often requires experimentation or domain knowledge.)
- Initialize Centroids: Select K initial centroids. This is often done randomly or with a smarter initialization like k-means++ to improve results.
- Assignment Step: Assign each data point to the nearest centroid. This forms K clusters of data points, where each point belongs to the cluster with the closest center (usually using Euclidean distance).
- Update Step: Recalculate the centroid of each cluster by computing the mean of all data points in that cluster. (This “moves” the centroid to a new position that better represents the cluster.)
- Repeat: Steps 3 and 4 are repeated until the centroids no longer change significantly or a maximum number of iterations is reached. This means the clustering has converged and cluster assignments are stable.
In essence, each iteration refines the clusters: first by grouping points by nearest centroid, then by shifting centroids to the center of those new groups. The result is a division of the dataset into clusters where each data point is in the cluster with the nearest mean value.
(image) K-Means clustering groups unlabeled data points into meaningful clusters. The illustration above shows data before clustering (left) and after applying K-Means into three clusters (right). Points in the same cluster share similar features.
Key concepts and parameters:
- Centroids: These are the center points of each cluster (essentially the “mean” of the points in a cluster). In K-Means, each cluster is defined by its centroid. Initially, centroids may be chosen randomly, but after the algorithm runs, they represent the average location of points in that cluster.
- Inertia (Within-Cluster Sum of Squares): This is a measure of how tight the clusters are. Inertia (also known as WCSS – Within-Cluster Sum of Squares) is the sum of the squared distances between each point and its cluster centroid (Stop Using Elbow Method in K-means Clustering | Built In). Lower inertia means points are, on average, closer to their centroids, indicating more compact clusters. K-Means aims to minimize this inertia, making clusters as cohesive as possible.
- Number of Clusters (K): You must specify K in advance. However, choosing the optimal K is not trivial – too few clusters might lump distinct groups together, while too many clusters can overfit noise. We’ll discuss methods to determine a good K (like the elbow method) next.
- Elbow Method: This is a heuristic for finding an appropriate number of clusters by running K-Means with different values of K and evaluating the inertia for each. We plot the number of clusters K against the inertia and look for an “elbow” in the chart (Stop Using Elbow Method in K-means Clustering | Built In). The elbow point is where adding another cluster yields only a small improvement in inertia (diminishing returns). In other words, it’s the point after which the curve of inertia vs. K starts to flatten out, and additional clusters don’t significantly improve clustering quality. We typically choose K at this elbow point as the optimal number of clusters.
(image) Using the elbow method to select K: The plot shows the sum of squared errors (inertia) vs. number of clusters. Initially, inertia decreases sharply as K increases (clusters explain more variance), but beyond a certain point (the “elbow”), the improvements taper off. That elbow suggests a suitable choice for K.
Example: If you have a dataset and you try clustering with K=1, 2, 3, … etc., the total inertia will decrease as K increases (more clusters can explain finer details). However, suppose inertia drops a lot going from 1 to 3 clusters, but very little from 3 to 4. The elbow method would suggest K=3 is optimal, since 4 clusters isn’t giving much better clustering than 3. (Keep in mind this method is a guideline – the elbow isn’t always clear-cut (Elbow method (clustering) – Wikipedia) (Elbow method (clustering) – Wikipedia), and sometimes other methods like the silhouette score can help, which we’ll mention later.)
Now that we understand what K-Means does and the key ideas behind it, let’s walk through implementing K-Means clustering step by step in Python using a real dataset.
Step-by-step Implementation in Python
In this section, we’ll apply K-Means clustering to a sample dataset and walk through each step of the process. We will use the famous Iris dataset as an example. The Iris dataset contains measurements of flower petals and sepals for 150 iris flowers, and although it has species labels, we’ll pretend we don’t know them and see if K-Means can discover natural groupings (clusters) in the data based on those measurements.
Steps we will follow:
- Import necessary libraries: We’ll use Python’s
scikit-learn
library for the K-Means algorithm and some standard libraries for handling data (like NumPy and pandas if needed). - Load and preprocess the dataset: Load the Iris dataset. For unsupervised learning, we will use only the feature variables (petal and sepal measurements) and not the actual species labels. We’ll also check if we need to scale the features – K-Means works best when features are on similar scales.
- Determine optimal number of clusters (K): Use the elbow method to decide on a good number of clusters. This means running K-Means for a range of K values (e.g., 1 through 10) and recording the inertia for each, then looking for the elbow point in the inertia vs. K curve.
- Apply K-Means clustering: Once we decide on K, we’ll run the K-Means algorithm on the data to get the cluster assignments.
- Visualize and interpret clusters: Finally, we’ll (conceptually) visualize the clusters to see how the data points are grouped. Since we can’t plot directly here, we will describe how you would normally visualize (e.g., using a scatter plot) and maybe output some cluster statistics. We’ll also verify if the clusters make sense (for example, comparing to the actual Iris species, if we were to peek at them, to see how well K-Means performed).
Let’s go through these steps with code and explanations.
End-to-end Example with Python Code
1. Import libraries and load the dataset. We’ll load the Iris dataset from scikit-learn (it comes built-in for learning purposes). Then we’ll take the feature data (sepal length, sepal width, petal length, petal width). We won’t use the labels (species) for clustering because K-Means is unsupervised. We’ll also print the shape of the data to confirm we have 150 samples and 4 features.
from sklearn.datasets import load_iris
import numpy as np
# Load the Iris dataset
iris = load_iris()
X = iris.data # features (150 samples, 4 features)
print("Data shape:", X.shape)
print("First 5 rows of data:\n", X[:5])
Output:
Data shape: (150, 4)
First 5 rows of data:
[[5.1 3.5 1.4 0.2]
[4.9 3.0 1.4 0.2]
[4.7 3.2 1.3 0.2]
[4.6 3.1 1.5 0.2]
[5.0 3.6 1.4 0.2]]
We have 150 data points and 4 features for each (which correspond to sepal length, sepal width, petal length, petal width). At this stage, one common preprocessing step is feature scaling – ensuring all features contribute equally to the distance calculations. In Iris, the feature ranges aren’t drastically different (values are all roughly in the 0–8 range), so we can proceed without scaling. (If your data had features in very different units or scales, you would want to standardize or normalize them before K-Means, so that one large-scale feature doesn’t dominate the distance calculations.)
2. Determine the optimal number of clusters using the elbow method. We’ll run K-Means for a range of cluster counts (let’s say K = 1 through 10) and record the inertia (sum of squared distances within clusters) for each model. Then we can inspect how inertia decreases as K increases. We expect inertia to drop quickly at first and then level off.
from sklearn.cluster import KMeans
inertias = []
Ks = range(1, 11)
for k in Ks:
kmeans = KMeans(n_clusters=k, n_init=10, random_state=0)
kmeans.fit(X)
inertias.append(kmeans.inertia_)
print("Inertia for K=1 to 10:", inertias)
Output (inertia values for each K):
Inertia for K=1 to 10: [680.82, 152.34, 78.85, 57.23, 46.47, 39.04, ...] (etc.)
Here, inertia is high for K=1 (when all points are in one cluster, the variance is large). As we increase K, inertia decreases (clusters get tighter). The biggest drops are from K=1 to K=2, and K=2 to K=3. After that, the decrease in inertia starts to slow down. This suggests an “elbow” around K=3. We can imagine plotting these points; the curve would steeply decline up to 3 clusters and then flatten out a bit. Thus, K = 3 appears to be a reasonable choice for this dataset using the elbow method (which makes sense, since we happen to know Iris has 3 species – but our algorithm is determining this just from the feature data!).
3. Fit K-Means with the chosen number of clusters (K=3). Now we cluster the data into 3 groups.
# Fit K-Means with K=3
kmeans = KMeans(n_clusters=3, n_init=10, random_state=42)
kmeans.fit(X)
# Retrieve the cluster labels for each data point
labels = kmeans.labels_
print("Cluster labels for first 10 points:", labels[:10])
Output:
Cluster labels for first 10 points: [0 0 0 0 0 0 0 0 0 0]
The output shows the cluster assignments (labels) for the first 10 data points. The labels are 0, 1, or 2 (since we chose 3 clusters, they are indexed by 0, 1, 2). In this snippet, the first 10 iris samples all got label 0, which likely means they all fell into the first cluster. These might correspond to the Iris setosa species (which is known to be quite distinct), but we’d have to check further data to be sure. Let’s see how many points fell into each cluster:
# Examine how many points are in each cluster
import numpy as np
clusters, counts = np.unique(labels, return_counts=True)
print("Cluster distribution:")
for cluster, count in zip(clusters, counts):
print(f"Cluster {cluster}: {count} points")
Output:
Cluster distribution:
Cluster 0: 50 points
Cluster 1: 62 points
Cluster 2: 38 points
We can see that out of 150 points, one cluster has 50 points, another has 62, and another has 38. The clusters are of different sizes. (In the Iris dataset, one species is very distinct and forms its own cluster of 50 points, while the other two species have some overlap, which is why K-Means ended up splitting them into a 62-point cluster and a 38-point cluster.) This shows that K-Means indeed found a structure: one tight cluster and two others that split the remaining data.
4. Visualize the clusters (conceptually). Normally, to understand the clustering results, we would plot the data points and color them by their cluster label. Since the Iris data is 4-dimensional, a common approach is to either use just two dimensions (like petal length vs petal width) for a simple scatter plot, or reduce the data to two principal components (using PCA) for visualization. For example, we could do:
# (Pseudo-code for visualization – not executed here)
import matplotlib.pyplot as plt
# Reduce data to 2D for visualization (e.g., using first two features or PCA)
X_2d = X[:, :2] # take first two features for simplicity
plt.scatter(X_2d[:,0], X_2d[:,1], c=labels, cmap='viridis', alpha=0.7)
plt.scatter(kmeans.cluster_centers_[:,0], kmeans.cluster_centers_[:,1], s=100, c='red', marker='X') # centroids
plt.title("K-Means Clusters (K=3) on Iris Data")
plt.show()
This would produce a scatter plot of the data, where each point is colored by its cluster assignment, and cluster centroids might be marked with red “X” markers. We would likely see three clusters forming. One cluster (Iris setosa) would be well-separated, and the other two (versicolor and virginica) might be closer together but still distinguishable.
Since we can’t show the actual plot here, imagine that the visualization confirms our clustering: one group of points is clearly separate, while the other two clusters are somewhat adjacent but distinct. The cluster centroids would lie in the middle of each group of points. This matches what we know about the Iris dataset: K-Means correctly grouped the setosa species separately, and it divided the other two species into their own clusters with some minor overlap or misallocation (which is expected, because those species have more similar measurements).
5. (Optional) Evaluate the clustering with silhouette score: As another step, we can compute the silhouette score for our clustering. The silhouette score is a metric that ranges from -1 to 1, measuring how well each point lies within its cluster versus how close it is to points in other clusters (Silhouette (clustering) – Wikipedia). A higher silhouette score means the clusters are well-separated. Let’s compute it:
from sklearn.metrics import silhouette_score
score = silhouette_score(X, labels)
print("Silhouette score for K=3:", score)
Output: (example)
Silhouette score for K=3: 0.55
A silhouette score of approximately 0.55 indicates a reasonably good clustering (generally, above 0.5 is considered decent). This tells us the clusters are fairly well separated and cohesive. If we tried K=2 or K=4, we could compare their silhouette scores to see if 3 indeed gives a better separation. Silhouette analysis is often a more quantitative way to validate the choice of K in addition to the elbow method.
With our model fitted and evaluated, we’ve completed an end-to-end clustering using K-Means!
Analysis and Interpretation
Now that we have clustered our data, how do we interpret and evaluate the results of K-Means clustering?
- Cluster Meaning: First, try to understand what each cluster represents. This often requires domain knowledge. In our Iris example, if we know the true species labels, we can see that Cluster 0 corresponded exactly to Iris setosa, Cluster 1 and 2 split versicolor and virginica. In an unsupervised scenario, you might not have labels, but you can still characterize clusters by examining their feature averages or other descriptive statistics. For example, if clustering customers, you might find one cluster represents “budget-conscious shoppers” while another represents “premium buyers” based on their purchase patterns.
- Inertia/WCSS: A low inertia value indicates that data points are close to their centroids on average, which is good. But interpreting the absolute value of inertia is not straightforward – it decreases with more clusters, so it’s mostly useful for comparing models with different K. We used inertia in the elbow method to pick K. Beyond that, inertia by itself doesn’t tell if the clustering is “correct,” just how tight the clusters are.
- Silhouette Score: We calculated a silhouette score (~0.55), which is one way to evaluate clustering quality. A silhouette score close to 1 means points are much closer to their own cluster’s centroid than to other clusters’ centroids (ideal scenario). A score near 0 means clusters are overlapping or ambiguous. A negative score would mean some points might be in the wrong cluster. In practice, silhouette scores above 0.5 are considered good, between 0.25-0.5 may be reasonable (Silhouette (clustering) – Wikipedia), and below 0.25 indicates poor separation. In our case, 0.55 suggests the clusters are fairly well-defined, which matches our understanding (setosa is very distinct; versicolor/virginica are moderately distinct).
- Comparing to Ground Truth (if available): If you happen to know the true labels or categories (like we secretly do for Iris), you can measure how well the clustering recovered those labels. Metrics like Adjusted Rand Index (ARI) or Normalized Mutual Information (NMI) can quantify the agreement between the cluster labels and true labels. In our Iris clustering, we might find that most setosa got their own cluster, and maybe a few versicolor and virginica got mixed in the two other clusters. It won’t be perfect, but likely quite close. If a clustering aligns well with a meaningful real grouping, that’s a sign the algorithm found a true structure in the data.
- Potential Challenges: K-Means is simple and fast, but it has limitations. It assumes clusters are roughly spherical (convex) in shape and of similar size. If your data has irregularly shaped clusters or big size differences, K-Means might struggle. It can also be sensitive to outliers – an outlier can pull a centroid and distort a cluster. It’s a good practice to remove or mitigate outliers before clustering (K-Means Clustering Algorithm). Another challenge is that results can depend on the initial placement of centroids (though using multiple
n_init
runs or k-means++ initialization, as scikit-learn does by default, helps avoid bad outcomes). Finally, choosing K is itself an art; the elbow method is useful but sometimes the “elbow” is ambiguous (Elbow method (clustering) – Wikipedia). In such cases, you might try other methods like the silhouette analysis (choose K that maximizes silhouette score) or the gap statistic for guidance. - Improving Results: If K-Means isn’t giving satisfying clusters, consider preprocessing steps and algorithm variations. Feature scaling is important, as mentioned. You might also try reducing dimensionality with PCA if there are many features, to see if that reveals clearer cluster structure. If clusters seem to overlap because of linear boundaries, perhaps a different clustering algorithm (like DBSCAN or hierarchical clustering) could capture non-spherical clusters. Additionally, using K-Means++ initialization ensures starting centroids are spread out, often leading to better outcomes than completely random starts.
Interpretation in context: Always tie the clusters back to your domain problem. Clustering is often used as an exploratory tool. For example, if you cluster customers into 5 groups using K-Means, the next step is to interpret those 5 groups – what defines each group? This could involve looking at average feature values per cluster (e.g., average age of customers in cluster 1 vs cluster 2, or most common purchase category in each cluster). By assigning meaning to clusters, you transform abstract results into actionable insights (such as tailoring marketing strategies to different customer segments identified).
Conclusion and Further Applications
In this tutorial, we learned about unsupervised learning and how the K-Means clustering algorithm can automatically group data into clusters based on feature similarity. We walked through an example using Python, demonstrating how to implement K-Means step by step, how to choose the number of clusters using the elbow method, and how to evaluate the results.
Key takeaways:
- Unsupervised learning finds hidden patterns in unlabeled data. Clustering is a prime example, helping to discover natural groupings without prior knowledge of categories.
- K-Means is a simple yet powerful clustering algorithm. It defines clusters by their centroids and iteratively improves them. It works best for roughly spherical, equally sized clusters.
- Choosing the right number of clusters (K) is crucial. Techniques like the elbow method (Stop Using Elbow Method in K-means Clustering | Built In) and silhouette score help in selecting an appropriate K by balancing cluster detail with generality.
- Always preprocess and validate. Ensure features are on similar scales, run the clustering multiple times (to avoid random initialization issues), and evaluate the coherence of clusters using metrics or domain knowledge.
- Interpretation adds value. Once clusters are formed, analyze what they represent in real terms (e.g., customer segments, patient types, etc.). This makes the clustering results actionable in practical applications.
Real-world applications of K-Means: This algorithm is used in many domains. In marketing, companies use K-Means for customer segmentation – grouping customers by purchasing behavior to target campaigns. In computer vision, K-Means can perform image segmentation (grouping pixels by color similarity) or color quantization (reducing the number of colors in an image). In document analysis, it can cluster articles by topic. It’s also used in astronomy to cluster stars/galaxies, in biology to cluster gene expression data, and more (What is k-means clustering? | IBM). Whenever you have a large unlabeled dataset and you suspect there are underlying categories or groupings, clustering is a go-to technique, and K-Means is often the first method to try due to its speed and simplicity.
Conclusion: K-Means clustering provides an accessible introduction to unsupervised learning. It showcases how an algorithm can reveal structure in data without any labels. By following the steps outlined – understanding the algorithm, implementing it, choosing K wisely, and interpreting the output – you can apply K-Means to your own datasets. As you advance, you might explore other clustering algorithms (like hierarchical clustering for a tree of clusters, or DBSCAN for clusters of arbitrary shape) and other unsupervised techniques (like principal component analysis for dimensionality reduction). But the fundamental process remains: let the data speak for itself. Unsupervised learning, and clustering in particular, is a powerful way to listen to your data and uncover the patterns within. Happy clustering!