Mastering PyTorch: 10 Essential Functions for Deep Learning
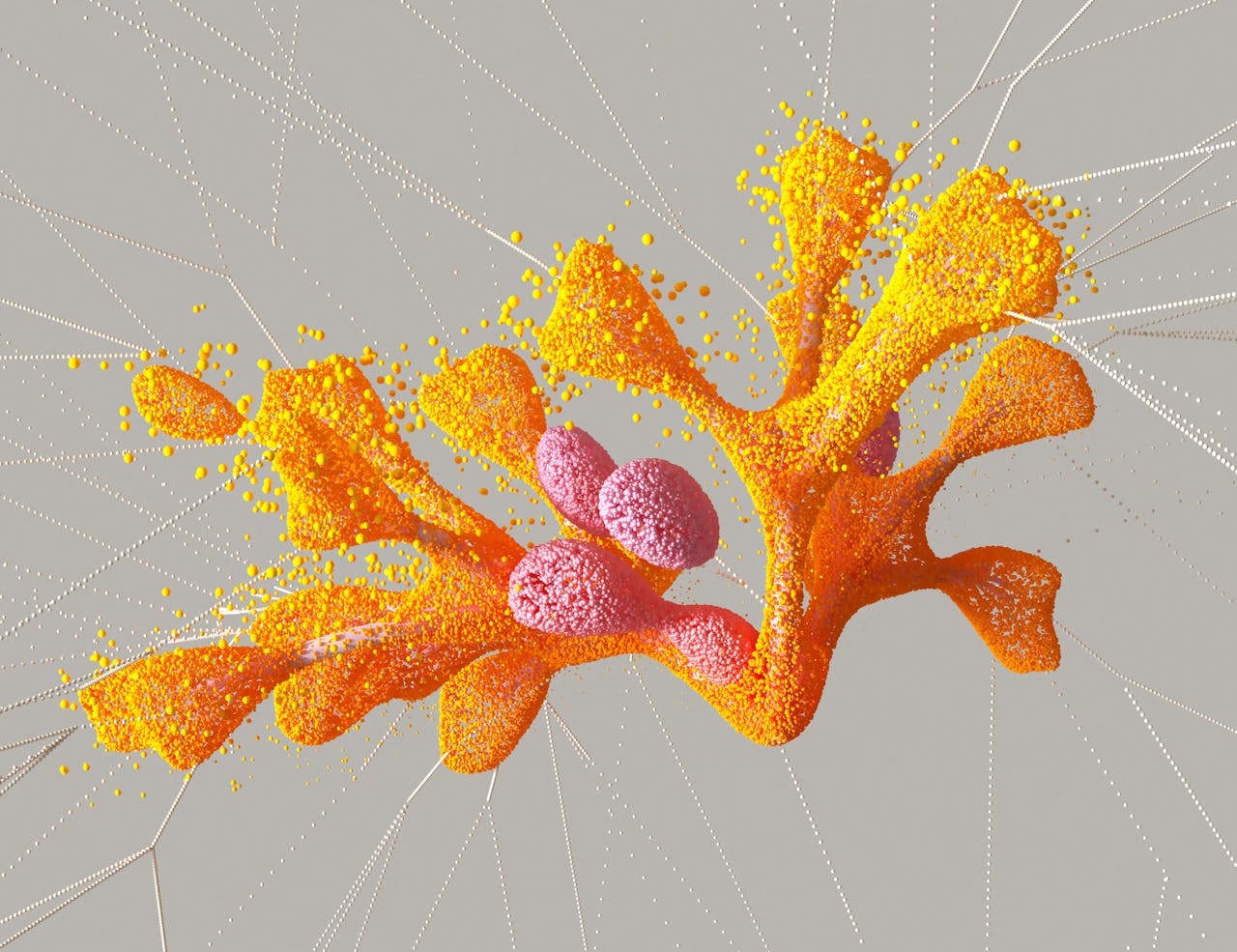
- 1. torch.tensor(): The Foundation of PyTorch Operations
- 2. torch.nn.Module: The Backbone of Neural Network Architecture
- 3. torch.optim: Optimizing Your Model's Performance
- 4. torch.nn.functional: A Treasure Trove of Activation Functions and Operations
- 5. torch.autograd: The Engine Behind Automatic Differentiation
- 6. torch.utils.data: Streamlining Data Handling for Efficient Training
- 7. torch.jit: Bridging the Gap Between Research and Production
- 8. torch.distributed: Scaling Up Your Deep Learning with Distributed Training
- 9. torch.cuda: Unleashing the Power of GPU Acceleration
- 10. torch.onnx: Bridging PyTorch with the Broader AI Ecosystem
PyTorch has become one of the most popular deep learning frameworks, thanks to its intuitive design, dynamic computational graphs, and extensive ecosystem. Whether you’re a beginner or an experienced practitioner, understanding the core functions of PyTorch is crucial for building efficient and powerful neural networks. In this comprehensive guide, we’ll explore the top 10 PyTorch functions that are indispensable for deep learning tasks. By mastering these functions, you’ll be well-equipped to tackle a wide range of machine learning challenges and optimize your models for better performance.
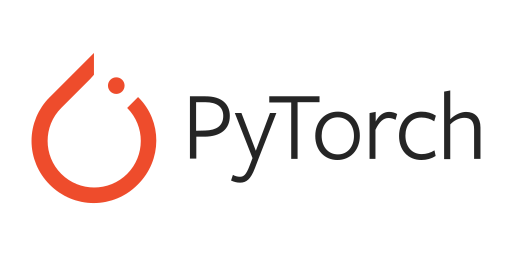
1. torch.tensor(): The Foundation of PyTorch Operations
At the heart of PyTorch lies the tensor, a fundamental data structure that serves as the building block for all neural network operations. The torch.tensor() function is your gateway to creating these multi-dimensional arrays, which can represent various types of data, from simple scalars to complex matrices.
Let’s explore the versatility of torch.tensor():
python
import torch
# Creating tensors from Python lists
scalar = torch.tensor(3.14)
vector = torch.tensor([1, 2, 3])
matrix = torch.tensor([[1, 2], [3, 4]])
# Creating tensors with specific data types
float_tensor = torch.tensor([1.0, 2.0, 3.0], dtype=torch.float32)
int_tensor = torch.tensor([1, 2, 3], dtype=torch.int64)
# Creating tensors on specific devices
cpu_tensor = torch.tensor([1, 2, 3], device='cpu')
gpu_tensor = torch.tensor([1, 2, 3], device='cuda:0') # Requires a CUDA-enabled GPU
# Creating tensors with specific shapes
zeros = torch.zeros(3, 4)
ones = torch.ones(2, 3, 5)
random = torch.rand(3, 3)
print("Scalar:", scalar)
print("Vector:", vector)
print("Matrix:", matrix)
print("Float Tensor:", float_tensor)
print("Integer Tensor:", int_tensor)
print("CPU Tensor:", cpu_tensor)
print("GPU Tensor:", gpu_tensor)
print("Zeros Tensor:", zeros)
print("Ones Tensor:", ones)
print("Random Tensor:", random)
Understanding the nuances of torch.tensor() is crucial because it allows you to:
- Convert data from various sources (lists, NumPy arrays, etc.) into PyTorch tensors.
- Specify the data type (dtype) of your tensors, which can impact computational efficiency and precision.
- Control the device (CPU or GPU) on which your tensors reside, enabling seamless GPU acceleration when available.
- Create tensors with specific shapes and initial values, which is essential for initializing neural network parameters.
By mastering torch.tensor(), you lay a solid foundation for all subsequent PyTorch operations and deep learning tasks.
2. torch.nn.Module: The Backbone of Neural Network Architecture
The torch.nn.Module class is the cornerstone of building neural networks in PyTorch. It provides a structured way to define layers, organize computations, and manage the flow of data through your model. By inheriting from nn.Module, you can create custom layers and entire network architectures with ease.
Let’s explore the power and flexibility of torch.nn.Module:
python
import torch
import torch.nn as nn
class SimpleNN(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(SimpleNN, self).__init__()
self.layer1 = nn.Linear(input_size, hidden_size)
self.activation = nn.ReLU()
self.layer2 = nn.Linear(hidden_size, output_size)
def forward(self, x):
x = self.layer1(x)
x = self.activation(x)
x = self.layer2(x)
return x
# Create an instance of the model
model = SimpleNN(input_size=10, hidden_size=20, output_size=5)
# Generate some random input data
input_data = torch.randn(1, 10)
# Forward pass through the model
output = model(input_data)
print("Model Architecture:")
print(model)
print("\nModel Output Shape:", output.shape)
# Accessing model parameters
for name, param in model.named_parameters():
print(f"\nParameter: {name}")
print(f"Shape: {param.shape}")
print(f"Requires Gradient: {param.requires_grad}")
The torch.nn.Module class offers several key benefits:
- Modular Design: You can create complex neural networks by combining simple building blocks, promoting code reusability and maintainability.
- Automatic Parameter Management: PyTorch automatically tracks and manages all parameters defined within nn.Module subclasses, making it easy to update them during training.
- Device Agnostic: Models built with nn.Module can be easily moved between CPU and GPU using the .to(device) method.
- Built-in Layers: PyTorch provides a wide range of pre-implemented layers (e.g., nn.Linear, nn.Conv2d, nn.LSTM) that you can use to construct your networks quickly.
- Customization: You can define custom forward passes and layer interactions, allowing for great flexibility in network design.
Understanding and leveraging torch.nn.Module is essential for creating scalable, maintainable, and efficient deep learning models in PyTorch.
3. torch.optim: Optimizing Your Model’s Performance
Optimization is a crucial aspect of training deep learning models, and PyTorch’s torch.optim module provides a comprehensive set of optimization algorithms to help you fine-tune your model’s parameters. These optimizers implement various strategies to update the model weights based on the computed gradients, aiming to minimize the loss function and improve the model’s performance.
Let’s explore some of the most popular optimizers and how to use them effectively:
python
import torch
import torch.nn as nn
import torch.optim as optim
# Define a simple model
class SimpleModel(nn.Module):
def __init__(self):
super(SimpleModel, self).__init__()
self.fc = nn.Linear(10, 1)
def forward(self, x):
return self.fc(x)
# Create model instance
model = SimpleModel()
# Generate some dummy data
X = torch.randn(100, 10)
y = torch.randn(100, 1)
# Define loss function
criterion = nn.MSELoss()
# Initialize different optimizers
sgd_optimizer = optim.SGD(model.parameters(), lr=0.01)
adam_optimizer = optim.Adam(model.parameters(), lr=0.01)
rmsprop_optimizer = optim.RMSprop(model.parameters(), lr=0.01)
# Training loop with different optimizers
optimizers = [sgd_optimizer, adam_optimizer, rmsprop_optimizer]
optimizer_names = ["SGD", "Adam", "RMSprop"]
for name, optimizer in zip(optimizer_names, optimizers):
print(f"\nTraining with {name} optimizer:")
for epoch in range(100):
# Forward pass
y_pred = model(X)
loss = criterion(y_pred, y)
# Backward pass and optimization
optimizer.zero_grad()
loss.backward()
optimizer.step()
if (epoch + 1) % 10 == 0:
print(f"Epoch [{epoch+1}/100], Loss: {loss.item():.4f}")
# Learning rate scheduling
scheduler = optim.lr_scheduler.StepLR(adam_optimizer, step_size=30, gamma=0.1)
print("\nTraining with learning rate scheduling:")
for epoch in range(100):
# Forward pass
y_pred = model(X)
loss = criterion(y_pred, y)
# Backward pass and optimization
adam_optimizer.zero_grad()
loss.backward()
adam_optimizer.step()
# Update learning rate
scheduler.step()
if (epoch + 1) % 10 == 0:
print(f"Epoch [{epoch+1}/100], Loss: {loss.item():.4f}, LR: {scheduler.get_last_lr()[0]:.6f}")
Key aspects of torch.optim:
- Variety of Algorithms: PyTorch offers a wide range of optimization algorithms, including SGD, Adam, RMSprop, and more, each with its own strengths and use cases.
- Customizable Hyperparameters: You can fine-tune optimizer behavior by adjusting learning rates, momentum, weight decay, and other algorithm-specific parameters.
- Parameter Groups: Optimizers allow you to specify different learning rates or hyperparameters for different parts of your model, enabling more granular control over the optimization process.
- Learning Rate Scheduling: PyTorch provides learning rate schedulers (torch.optim.lr_scheduler) that can automatically adjust the learning rate during training, helping to improve convergence and model performance.
- Gradient Clipping: Some optimizers support gradient clipping, which can help prevent exploding gradients and stabilize training in certain scenarios.
Understanding and effectively using torch.optim is crucial for:
- Achieving faster convergence during training
- Avoiding local minima and saddle points
- Handling different types of neural network architectures and datasets
- Fine-tuning model performance for specific tasks
By mastering the various optimizers and their configurations, you can significantly improve your model’s training efficiency and overall performance.
4. torch.nn.functional: A Treasure Trove of Activation Functions and Operations
The torch.nn.functional module, often imported as F, is a powerhouse of neural network operations, activation functions, and other essential components for building and training deep learning models. This module provides functional interfaces to many of the operations available in torch.nn, allowing for more flexibility in certain scenarios.
Let’s explore some of the key functions and their applications:
python
import torch
import torch.nn.functional as F
# Generate some sample data
x = torch.randn(5, 10)
target = torch.randint(0, 2, (5,)).float()
# Activation functions
relu_output = F.relu(x)
sigmoid_output = F.sigmoid(x)
tanh_output = F.tanh(x)
print("ReLU output:", relu_output)
print("Sigmoid output:", sigmoid_output)
print("Tanh output:", tanh_output)
# Softmax and log softmax
softmax_output = F.softmax(x, dim=1)
log_softmax_output = F.log_softmax(x, dim=1)
print("\nSoftmax output:", softmax_output)
print("Log Softmax output:", log_softmax_output)
# Loss functions
bce_loss = F.binary_cross_entropy(F.sigmoid(x[:, 0]), target)
mse_loss = F.mse_loss(x[:, 0], target)
print("\nBinary Cross Entropy Loss:", bce_loss.item())
print("Mean Squared Error Loss:", mse_loss.item())
# Pooling operations
max_pool = F.max_pool2d(torch.randn(1, 3, 32, 32), kernel_size=2, stride=2)
avg_pool = F.avg_pool2d(torch.randn(1, 3, 32, 32), kernel_size=2, stride=2)
print("\nMax Pooling output shape:", max_pool.shape)
print("Average Pooling output shape:", avg_pool.shape)
# Dropout
dropout_output = F.dropout(x, p=0.5, training=True)
print("\nDropout output:", dropout_output)
# Convolution operation
conv_input = torch.randn(1, 3, 32, 32)
conv_weight = torch.randn(16, 3, 3, 3)
conv_output = F.conv2d(conv_input, conv_weight, padding=1)
print("\nConvolution output shape:", conv_output.shape)
Key aspects of torch.nn.functional:
- Activation Functions: Provides a wide range of activation functions like ReLU, Sigmoid, Tanh, and more, which are essential for introducing non-linearity in neural networks.
- Loss Functions: Offers various loss functions such as Binary Cross Entropy, Mean Squared Error, and Cross Entropy Loss, which are crucial for defining the optimization objective.
- Pooling Operations: Includes max pooling and average pooling functions, which are commonly used in convolutional neural networks for downsampling and feature extraction.
- Normalization: Provides batch normalization and layer normalization functions, which help in stabilizing the learning process and reducing internal covariate shift.
- Dropout: Implements dropout, a regularization technique that helps prevent overfitting by randomly zeroing out a portion of neurons during training.
- Convolution Operations: Offers various convolution functions (1D, 2D, 3D) that are fundamental to convolutional neural networks.
- Utility Functions: Includes functions like softmax, log_softmax, and one_hot, which are often used in preprocessing or post-processing steps.
Understanding and effectively using torch.nn.functional is crucial for:
- Implementing custom layers and operations in your neural networks
- Applying activation functions and loss functions in a more flexible manner
- Performing operations on tensors that don’t require learnable parameters
- Experimenting with different network architectures and components
By mastering the functions in torch.nn.functional, you gain the flexibility to create more complex and customized neural network architectures, allowing you to tackle a wider range of deep learning problems effectively.
5. torch.autograd: The Engine Behind Automatic Differentiation
The torch.autograd module is the powerhouse that enables automatic differentiation in PyTorch, a crucial feature for training neural networks. This module tracks operations performed on tensors and automatically computes gradients during the backward pass. Understanding how to work with torch.autograd is essential for implementing custom loss functions, creating complex computational graphs, and debugging gradient flow in your models.
Let’s explore the key components and functionalities of torch.autograd:
python
import torch
# Creating tensors with gradient tracking
x = torch.tensor([1.0, 2.0, 3.0], requires_grad=True)
y = torch.tensor([4.0, 5.0, 6.0], requires_grad=True)
# Performing operations
z = x * y
w = z.sum()
print("x:", x)
print("y:", y)
print("z:", z)
print("w:", w)
# Computing gradients
w.backward()
print("\nGradients:")
print("x.grad:", x.grad)
print("y.grad:", y.grad)
# Using torch.autograd.grad for higher-order derivatives
x = torch.tensor([2.0], requires_grad=True)
y = x * x * x
first_order = torch.autograd.grad(y, x, create_graph=True)[0]
print("\nFirst-order derivative:", first_order)
second_order = torch.autograd.grad(first_order, x)[0]
print("Second-order derivative:", second_order)
# Custom autograd function
class CustomExp(torch.autograd.Function):
@staticmethod
def forward(ctx, x):
result = torch.exp(x)
ctx.save_for_backward(result)
return result
@staticmethod
def backward(ctx, grad_output):
result, = ctx.saved_tensors
return grad_output * result
# Using the custom autograd function
x = torch.tensor([1.0, 2.0, 3.0], requires_grad=True)
custom_exp = CustomExp.apply
y = custom_exp(x)
z = y.sum()
z.backward()
print("\nCustom autograd function:")
print("x:", x)
print("y:", y)
print("x.grad:", x.grad)
# Gradient checkpointing for memory efficiency
def expensive_function(x):
# Simulate an expensive computation
for _ in range(1000):
x = x * x
return x
x = torch.tensor([2.0], requires_grad=True)
y = torch.utils.checkpoint.checkpoint(expensive_function, x)
z = y.sum()
z.backward()
print("\nGradient checkpointing:")
print("x:", x)
print("y:", y)
print("x.grad:", x.grad)
Key aspects of torch.autograd:
- Automatic Differentiation: PyTorch uses a technique called reverse-mode autodiff, which efficiently computes gradients for all parameters in a single backward pass.
- Dynamic Computation Graphs: Unlike static frameworks, PyTorch builds the computation graph dynamically, allowing for more flexible and intuitive model designs.
- Higher-Order Derivatives: torch.autograd.grad allows you to compute higher-order derivatives, which can be useful for certain optimization techniques and scientific computing applications.
- Custom Autograd Functions: You can define custom autograd functions with forward and backward methods, enabling you to extend PyTorch’s capabilities and implement novel operations with automatic gradient computation.
- Gradient Checkpointing: This technique allows you to trade computation for memory, which is particularly useful when training very deep networks or working with limited GPU memory.
Understanding and effectively using torch.autograd is crucial for:
– Implementing custom loss functions and regularization techniques
– Debugging gradient flow in complex neural network architectures
– Developing novel optimization algorithms that require access to gradient information
– Implementing advanced machine learning techniques like meta-learning and neural architecture search
By mastering torch.autograd, you gain the ability to create more sophisticated and efficient deep learning models, push the boundaries of what’s possible with neural networks, and tackle complex optimization problems in various domains.
6. torch.utils.data: Streamlining Data Handling for Efficient Training
The torch.utils.data module is a powerful tool for managing datasets and creating efficient data loading pipelines in PyTorch. It provides classes and functions that help you organize your data, create batches, and iterate through your dataset during training. Proper use of this module can significantly improve your model’s training speed and memory efficiency.
Let’s explore the key components and functionalities of torch.utils.data:
import torch
from torch.utils.data import Dataset, DataLoader
import numpy as np
# Custom Dataset class
class CustomDataset(Dataset):
def __init__(self, size):
self.data = torch.randn(size, 10)
self.labels = torch.randint(0, 2, (size,))
def __len__(self):
return len(self.data)
def __getitem__(self, idx):
return self.data[idx], self.labels[idx]
# Create dataset and dataloader
dataset = CustomDataset(1000)
dataloader = DataLoader(dataset, batch_size=32, shuffle=True, num_workers=4)
# Iterate through the dataloader
for batch_idx, (data, labels) in enumerate(dataloader):
print(f"Batch {batch_idx + 1}:")
print("Data shape:", data.shape)
print("Labels shape:", labels.shape)
print("First few labels:", labels[:5])
print()
if batch_idx == 2: # Print only first 3 batches
break
# Using transforms
from torchvision import transforms
transform = transforms.Compose([
transforms.ToTensor(),
transforms.Normalize((0.5,), (0.5,))
])
class TransformedDataset(Dataset):
def __init__(self, size, transform=None):
self.data = np.random.rand(size, 28, 28) # Simulating image data
self.labels = torch.randint(0, 10, (size,))
self.transform = transform
def __len__(self):
return len(self.data)
def __getitem__(self, idx):
image = self.data[idx]
label = self.labels[idx]
if self.transform:
image = self.transform(image)
return image, label
transformed_dataset = TransformedDataset(1000, transform=transform)
transformed_dataloader = DataLoader(transformed_dataset, batch_size=32, shuffle=True)
# Demonstrate transformed data
for data, labels in transformed_dataloader:
print("Transformed data shape:", data.shape)
print("Data range:", data.min().item(), "to", data.max().item())
break
# Using sampler for imbalanced datasets
from torch.utils.data import WeightedRandomSampler
class ImbalancedDataset(Dataset):
def __init__(self, size):
self.data = torch.randn(size, 10)
self.labels = torch.cat([torch.zeros(int(size * 0.9)), torch.ones(int(size * 0.1))])
def __len__(self):
return len(self.data)
def __getitem__(self, idx):
return self.data[idx], self.labels[idx]
imbalanced_dataset = ImbalancedDataset(1000)
# Calculate weights for each sample
class_sample_count = torch.tensor(
[(imbalanced_dataset.labels == t).sum() for t in torch.unique(imbalanced_dataset.labels, sorted=True)]
)
weight = 1. / class_sample_count.float()
samples_weight = torch.tensor([weight[t] for t in imbalanced_dataset.labels])
sampler = WeightedRandomSampler(samples_weight, len(samples_weight))
imbalanced_dataloader = DataLoader(imbalanced_dataset, batch_size=32, sampler=sampler)
# Check class distribution in batches
class_counts = {0: 0, 1: 0}
for _ in range(10): # Check 10 batches
_, labels = next(iter(imbalanced_dataloader))
class_counts[0] += (labels == 0).sum().item()
class_counts[1] += (labels == 1).sum().item()
print("\nClass distribution after using WeightedRandomSampler:")
print(f"Class 0: {class_counts[0]}, Class 1: {class_counts[1]}")
Key aspects of torch.utils.data:
- Dataset Class: This is the foundation for creating custom datasets. By inheriting from torch.utils.data.Dataset and implementing len and getitem methods, you can easily create datasets that work seamlessly with PyTorch’s data loading utilities.
- DataLoader: This class provides an iterable over the dataset and handles batching, shuffling, and parallel data loading. It’s highly customizable, allowing you to control batch size, shuffling behavior, number of worker processes, and more.
- Transforms: While not directly part of torch.utils.data, the transforms module (especially in torchvision) works hand-in-hand with datasets to apply data augmentation and preprocessing on-the-fly.
- Samplers: Custom sampling strategies can be implemented using samplers, which are particularly useful for handling imbalanced datasets or implementing curriculum learning.
- Collate Functions: You can define custom collate_fn to control how individual samples are combined into a batch, which is useful for handling variable-length sequences or complex data structures.
Understanding and effectively using torch.utils.data is crucial for:
- Efficiently loading and preprocessing large datasets
- Implementing custom datasets for unique data formats or sources
- Applying data augmentation techniques to improve model generalization
- Handling imbalanced datasets through custom sampling strategies
- Optimizing memory usage and training speed through proper batching and parallel data loading
By mastering torch.utils.data, you can significantly improve the efficiency of your deep learning workflows, handle a wide variety of data types and formats, and implement advanced training techniques that rely on custom data loading strategies.
7. torch.jit: Bridging the Gap Between Research and Production
torch.jit, also known as TorchScript, is a powerful tool in the PyTorch ecosystem that allows you to create serializable and optimizable models. It bridges the gap between eager mode development (which is great for research and experimentation) and graph-based execution (which is often necessary for production deployment). TorchScript enables you to export your models to a format that can be run in high-performance environments such as C++ runtimes.
Let’s explore the key components and functionalities of torch.jit:
python
import torch
import torch.nn as nn
# Define a simple model
class SimpleModel(nn.Module):
def __init__(self):
super(SimpleModel, self).__init__()
self.fc1 = nn.Linear(10, 20)
self.relu = nn.ReLU()
self.fc2 = nn.Linear(20, 1)
def forward(self, x):
x = self.fc1(x)
x = self.relu(x)
x = self.fc2(x)
return x
# Create an instance of the model
model = SimpleModel()
# Example input
example_input = torch.randn(1, 10)
# Tracing
traced_model = torch.jit.trace(model, example_input)
print("Traced Model:")
print(traced_model.graph)
# Scripting
scripted_model = torch.jit.script(model)
print("\nScripted Model:")
print(scripted_model.graph)
# Using the scripted model
output = scripted_model(example_input)
print("\nModel Output:", output)
# Saving and loading
torch.jit.save(scripted_model, "scripted_model.pt")
loaded_model = torch.jit.load("scripted_model.pt")
# Custom TorchScript function
@torch.jit.script
def custom_relu(x):
return torch.where(x > 0, x, torch.zeros_like(x))
# Using the custom function in a model
class CustomModel(nn.Module):
def __init__(self):
super(CustomModel, self).__init__()
self.fc = nn.Linear(10, 1)
def forward(self, x):
x = self.fc(x)
return custom_relu(x)
custom_model = CustomModel()
scripted_custom_model = torch.jit.script(custom_model)
print("\nCustom Model with TorchScript function:")
print(scripted_custom_model.graph)
# Handling control flow
@torch.jit.script
def control_flow_example(x, y):
if x.sum() > y.sum():
return x
else:
return y
print("\nControl Flow Example:")
print(control_flow_example.graph)
# Optimizing the model
with torch.jit.optimized_execution(True):
optimized_output = scripted_model(example_input)
print("\nOptimized Model Output:", optimized_output)
# Freezing the model
frozen_model = torch.jit.freeze(scripted_model)
print("\nFrozen Model Graph:")
print(frozen_model.graph)
Key aspects of torch.jit:
- Tracing: This method captures the computational graph by running an example input through the model. It’s fast and works well for models with static control flow.
- Scripting: This method analyzes the Python source code of your model and converts it to TorchScript. It can handle dynamic control flow and is more flexible than tracing.
- TorchScript Functions: You can define custom TorchScript functions using the @torch.jit.script decorator, allowing you to incorporate complex logic into your models.
- Saving and Loading: TorchScript models can be easily saved to disk and loaded in other environments, including C++ applications.
- Optimization: TorchScript enables various optimizations that can improve model performance, especially when running on different hardware or in production environments.
- Control Flow Handling: TorchScript can capture and optimize control flow structures like if statements and loops, making it possible to script models with dynamic behavior.
- Model Freezing: This feature allows you to convert model parameters to constants, which can lead to further optimizations and smaller model sizes.
Understanding and effectively using torch.jit is crucial for:
- Deploying PyTorch models in production environments, especially those that require high performance or integration with C++ codebases.
- Optimizing models for inference on various hardware platforms, including mobile devices and edge computing environments.
- Ensuring consistency between training and inference implementations, especially when dealing with complex model architectures.
- Enabling advanced optimizations that aren’t possible in eager mode execution.
- Creating portable model representations that can be easily shared and deployed across different systems.
By mastering torch.jit, you can bridge the gap between research prototypes and production-ready models, unlock performance optimizations, and deploy your PyTorch models in a wide range of environments and applications.
8. torch.distributed: Scaling Up Your Deep Learning with Distributed Training
As deep learning models and datasets continue to grow in size and complexity, distributed training has become increasingly important. The torch.distributed module provides tools and primitives for distributing your model across multiple GPUs or even multiple machines, allowing you to tackle larger problems and reduce training time.
Let’s explore the key components and functionalities of torch.distributed:
python
import torch
import torch.nn as nn
import torch.distributed as dist
import torch.multiprocessing as mp
from torch.nn.parallel import DistributedDataParallel as DDP
from torch.utils.data.distributed import DistributedSampler
from torch.utils.data import DataLoader
# Define a simple model
class SimpleModel(nn.Module):
def __init__(self):
super(SimpleModel, self).__init__()
self.fc = nn.Linear(10, 1)
def forward(self, x):
return self.fc(x)
def setup(rank, world_size):
dist.init_process_group("gloo", rank=rank, world_size=world_size)
def cleanup():
dist.destroy_process_group()
def train(rank, world_size):
setup(rank, world_size)
# Create model and move it to GPU with id rank
model = SimpleModel().to(rank)
ddp_model = DDP(model, device_ids=[rank])
loss_fn = nn.MSELoss()
optimizer = torch.optim.SGD(ddp_model.parameters(), lr=0.001)
# Create a dummy dataset
dataset = torch.utils.data.TensorDataset(
torch.randn(100, 10),
torch.randn(100, 1)
)
# Use DistributedSampler to handle data distribution
sampler = DistributedSampler(dataset, num_replicas=world_size, rank=rank)
dataloader = DataLoader(dataset, batch_size=10, sampler=sampler)
# Training loop
for epoch in range(10):
ddp_model.train()
sampler.set_epoch(epoch)
for batch, (data, target) in enumerate(dataloader):
data, target = data.to(rank), target.to(rank)
optimizer.zero_grad()
output = ddp_model(data)
loss = loss_fn(output, target)
loss.backward()
optimizer.step()
if rank == 0 and batch % 10 == 0:
print(f"Epoch {epoch}, Batch {batch}, Loss: {loss.item()}")
cleanup()
def run_demo(demo_fn, world_size):
mp.spawn(demo_fn,
args=(world_size,),
nprocs=world_size,
join=True)
if __name__ == "__main__":
world_size = 2 # Number of processes to spawn
run_demo(train, world_size)
Key aspects of torch.distributed:
- Process Groups: torch.distributed uses process groups to manage communication between different processes. The init_process_group function is used to initialize the distributed environment.
- DistributedDataParallel (DDP): This wrapper allows you to easily parallelize your model across multiple GPUs or machines. It automatically handles gradient synchronization and optimization.
- DistributedSampler: This sampler ensures that each process works on a different subset of the data, preventing data overlap between processes.
- Communication Primitives: torch.distributed provides various primitives like all_reduce, broadcast, and gather for synchronizing data between processes.
- Multi-GPU and Multi-Node Support: You can scale your training from multiple GPUs on a single machine to multiple machines in a cluster.
- Different Backend Support: PyTorch supports various backends for distributed training, including NCCL (optimized for NVIDIA GPUs), Gloo, and MPI.
Understanding and effectively using torch.distributed is crucial for:
- Scaling up your models to train on large datasets that don’t fit on a single GPU
- Reducing training time by parallelizing computation across multiple GPUs or machines
- Implementing advanced distributed algorithms like model parallelism or pipeline parallelism
- Optimizing resource utilization in large-scale deep learning projects
- Preparing your models for deployment in distributed inference scenarios
By mastering torch.distributed, you can tackle larger and more complex deep learning problems, significantly reduce training times, and efficiently utilize computational resources across multiple GPUs and machines.
9. torch.cuda: Unleashing the Power of GPU Acceleration
The torch.cuda module is your gateway to harnessing the immense computational power of NVIDIA GPUs for deep learning tasks. This module provides tools and utilities for managing GPU memory, controlling device allocation, and optimizing GPU performance. Understanding torch.cuda is crucial for efficiently utilizing GPU resources and achieving maximum speedup in your deep learning workflows.
Let’s explore the key components and functionalities of torch.cuda:
python
import torch
import torch.nn as nn
# Check CUDA availability
print("CUDA available:", torch.cuda.is_available())
print("CUDA version:", torch.version.cuda)
print("Number of CUDA devices:", torch.cuda.device_count())
# Get current device
current_device = torch.cuda.current_device()
print("Current CUDA device:", current_device)
# Device properties
device_props = torch.cuda.get_device_properties(current_device)
print("\nCurrent device properties:")
print(" Name:", device_props.name)
print(" Total memory:", device_props.total_memory / 1e9, "GB")
print(" CUDA capability:", f"{device_props.major}.{device_props.minor}")
# Moving tensors to GPU
cpu_tensor = torch.randn(1000, 1000)
gpu_tensor = cpu_tensor.cuda() # or cpu_tensor.to('cuda')
print("\nTensor device:", gpu_tensor.device)
# Creating tensors directly on GPU
gpu_tensor_direct = torch.randn(1000, 1000, device='cuda')
# Simple model on GPU
class SimpleModel(nn.Module):
def __init__(self):
super(SimpleModel, self).__init__()
self.fc = nn.Linear(100, 10)
def forward(self, x):
return self.fc(x)
model = SimpleModel().cuda()
print("\nModel device:", next(model.parameters()).device)
# GPU memory management
print("\nGPU memory usage:")
print(" Allocated:", torch.cuda.memory_allocated() / 1e9, "GB")
print(" Cached:", torch.cuda.memory_reserved() / 1e9, "GB")
# Manual memory management
large_tensor = torch.randn(10000, 10000, device='cuda')
print("After allocating large tensor:")
print(" Allocated:", torch.cuda.memory_allocated() / 1e9, "GB")
del large_tensor
torch.cuda.empty_cache()
print("After deleting and emptying cache:")
print(" Allocated:", torch.cuda.memory_allocated() / 1e9, "GB")
print(" Cached:", torch.cuda.memory_reserved() / 1e9, "GB")
# Using multiple GPUs
if torch.cuda.device_count() > 1:
print("\nUsing DataParallel for multi-GPU:")
model = nn.DataParallel(SimpleModel())
print("Model is now on:", next(model.parameters()).device)
# CUDA streams
stream1 = torch.cuda.Stream()
stream2 = torch.cuda.Stream()
with torch.cuda.stream(stream1):
tensor1 = torch.randn(1000, 1000, device='cuda')
torch.cuda.current_stream().synchronize()
with torch.cuda.stream(stream2):
tensor2 = torch.randn(1000, 1000, device='cuda')
torch.cuda.current_stream().synchronize()
print("\nCUDA streams synchronized")
# Benchmarking GPU operations
start_event = torch.cuda.Event(enable_timing=True)
end_event = torch.cuda.Event(enable_timing=True)
start_event.record()
result = torch.matmul(gpu_tensor, gpu_tensor)
end_event.record()
torch.cuda.synchronize()
print(f"\nMatrix multiplication time: {start_event.elapsed_time(end_event):.2f} ms")
# Pinned memory for faster CPU-GPU transfer
pinned_tensor = torch.randn(1000, 1000).pin_memory()
start_event.record()
pinned_tensor = pinned_tensor.cuda(non_blocking=True)
end_event.record()
torch.cuda.synchronize()
print(f"Transfer time (pinned memory): {start_event.elapsed_time(end_event):.2f} ms")
# Asynchronous GPU copy
async_tensor = torch.randn(1000, 1000, device='cuda')
cpu_tensor = torch.empty(1000, 1000)
start_event.record()
cpu_tensor.copy_(async_tensor, non_blocking=True)
end_event.record()
torch.cuda.synchronize()
print(f"Asynchronous copy time: {start_event.elapsed_time(end_event):.2f} ms")
Key aspects of torch.cuda:
- Device Management: torch.cuda provides functions to check CUDA availability, get device properties, and manage multiple GPUs.
- Memory Management: You can monitor and control GPU memory usage, including allocating, freeing, and caching memory.
- Data Transfer: The module offers efficient ways to move data between CPU and GPU, including pinned memory and asynchronous transfers.
- CUDA Streams: Streams allow for concurrent execution of operations on the GPU, potentially improving overall performance.
- Multi-GPU Support: torch.cuda facilitates the use of multiple GPUs through DataParallel and DistributedDataParallel.
- Benchmarking and Profiling: CUDA events can be used to accurately time GPU operations and identify performance bottlenecks.
- Asynchronous Execution: Many CUDA operations can be performed asynchronously, allowing for better overlap of computation and data transfer.
Understanding and effectively using torch.cuda is crucial for:
- Maximizing the performance of your deep learning models on GPU hardware
- Efficiently managing GPU memory to avoid out-of-memory errors in large models
- Implementing advanced techniques like model parallelism and pipeline parallelism
- Optimizing data transfer between CPU and GPU to reduce training and inference times
- Debugging and profiling GPU-accelerated PyTorch code
By mastering torch.cuda, you can fully leverage the power of GPU acceleration, handle larger models and datasets, and significantly speed up your deep learning workflows.
10. torch.onnx: Bridging PyTorch with the Broader AI Ecosystem
The torch.onnx module is a powerful tool that allows you to export your PyTorch models to the Open Neural Network Exchange (ONNX) format. ONNX is an open format designed to represent machine learning models, enabling interoperability between different frameworks. By converting your PyTorch models to ONNX, you can deploy them in a wide range of environments and leverage optimizations provided by various runtime engines.
Let’s explore the key components and functionalities of torch.onnx:
python
import torch
import torch.nn as nn
import torch.onnx
# Define a simple model
class SimpleModel(nn.Module):
def __init__(self):
super(SimpleModel, self).__init__()
self.conv1 = nn.Conv2d(1, 32, 3, 1)
self.conv2 = nn.Conv2d(32, 64, 3, 1)
self.fc1 = nn.Linear(9216, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = self.conv1(x)
x = nn.functional.relu(x)
x = self.conv2(x)
x = nn.functional.relu(x)
x = nn.functional.max_pool2d(x, 2)
x = torch.flatten(x, 1)
x = self.fc1(x)
x = nn.functional.relu(x)
x = self.fc2(x)
return x
# Instantiate the model
model = SimpleModel()
# Create a dummy input tensor
dummy_input = torch.randn(1, 1, 28, 28)
# Export the model to ONNX
torch.onnx.export(model, # model being run
dummy_input, # model input (or a tuple for multiple inputs)
"simple_model.onnx", # where to save the model (can be a file or file-like object)
export_params=True, # store the trained parameter weights inside the model file
opset_version=10, # the ONNX version to export the model to
do_constant_folding=True, # whether to execute constant folding for optimization
input_names = ['input'], # the model's input names
output_names = ['output'], # the model's output names
dynamic_axes={'input' : {0 : 'batch_size'}, # variable length axes
'output' : {0 : 'batch_size'}})
print("Model exported to ONNX format")
# Verify the exported model using ONNX Runtime
import onnx
import onnxruntime
# Load the ONNX model
onnx_model = onnx.load("simple_model.onnx")
# Check that the model is well formed
onnx.checker.check_model(onnx_model)
print("ONNX model is well formed")
# Create an ONNX Runtime session
ort_session = onnxruntime.InferenceSession("simple_model.onnx")
# Run the model in ONNX Runtime
ort_inputs = {ort_session.get_inputs()[0].name: dummy_input.numpy()}
ort_outputs = ort_session.run(None, ort_inputs)
# Compare ONNX Runtime and PyTorch results
torch_out = model(dummy_input)
np.testing.assert_allclose(torch_out.detach().numpy(), ort_outputs[0], rtol=1e-03, atol=1e-05)
print("Exported model has been tested with ONNXRuntime, and the result looks good!")
# Demonstrate ONNX model optimization
from onnxruntime.transformers import optimizer
from onnxruntime.transformers.onnx_model_bert import BertOptimizationOptions
opt_options = BertOptimizationOptions('bert')
opt_model = optimizer.optimize_model(
"simple_model.onnx",
'bert',
num_heads=12,
hidden_size=768,
optimization_options=opt_options)
opt_model.save_model_to_file("optimized_simple_model.onnx")
print("ONNX model optimized and saved")
# Load and run the optimized model
ort_session_opt = onnxruntime.InferenceSession("optimized_simple_model.onnx")
ort_outputs_opt = ort_session_opt.run(None, ort_inputs)
np.testing.assert_allclose(ort_outputs[0], ort_outputs_opt[0], rtol=1e-03, atol=1e-05)
print("Optimized model produces the same output as the original model")
Key aspects of torch.onnx:
- Model Export: The torch.onnx.export function allows you to convert PyTorch models to ONNX format, specifying various options like opset version and input/output names.
- Compatibility: ONNX supports a wide range of operators and model architectures, allowing you to export most PyTorch models.
- Dynamic Shapes: You can specify dynamic axes in your model, enabling flexibility in input shapes during inference.
- Optimization: ONNX models can be further optimized using tools like ONNX Runtime, potentially improving inference performance.
- Interoperability: Exported ONNX models can be run in various environments, including cloud services, edge devices, and different deep learning frameworks.
- Verification: You can use tools like ONNX Runtime to verify that the exported model produces the same results as the original PyTorch model.
Understanding and effectively using torch.onnx is crucial for:
- Deploying PyTorch models in production environments that use ONNX Runtime or other ONNX-compatible inference engines
- Leveraging hardware-specific optimizations provided by different ONNX runtimes
- Sharing models with collaborators who may be using different deep learning frameworks
- Integrating PyTorch models into larger AI pipelines that involve multiple frameworks or tools
- Optimizing model performance for inference in resource-constrained environments
By mastering torch.onnx, you can extend the reach of your PyTorch models beyond the PyTorch ecosystem, enabling deployment in a wide range of environments and leveraging optimizations provided by various ONNX-compatible runtimes.
In conclusion, these 10 essential PyTorch functions and modules – torch.tensor, torch.nn.Module, torch.optim, torch.nn.functional, torch.autograd, torch.utils.data, torch.jit, torch.distributed, torch.cuda, and torch.onnx – form the backbone of efficient and powerful deep learning development in PyTorch. By mastering these tools, you’ll be well-equipped to tackle a wide range of machine learning challenges, from research prototypes to production-ready models. Each of these components plays a crucial role in the PyTorch ecosystem, enabling you to build, train, optimize, and deploy state-of-the-art deep learning models across various platforms and environments.